Building a Multi-language App with Next.js and i18next
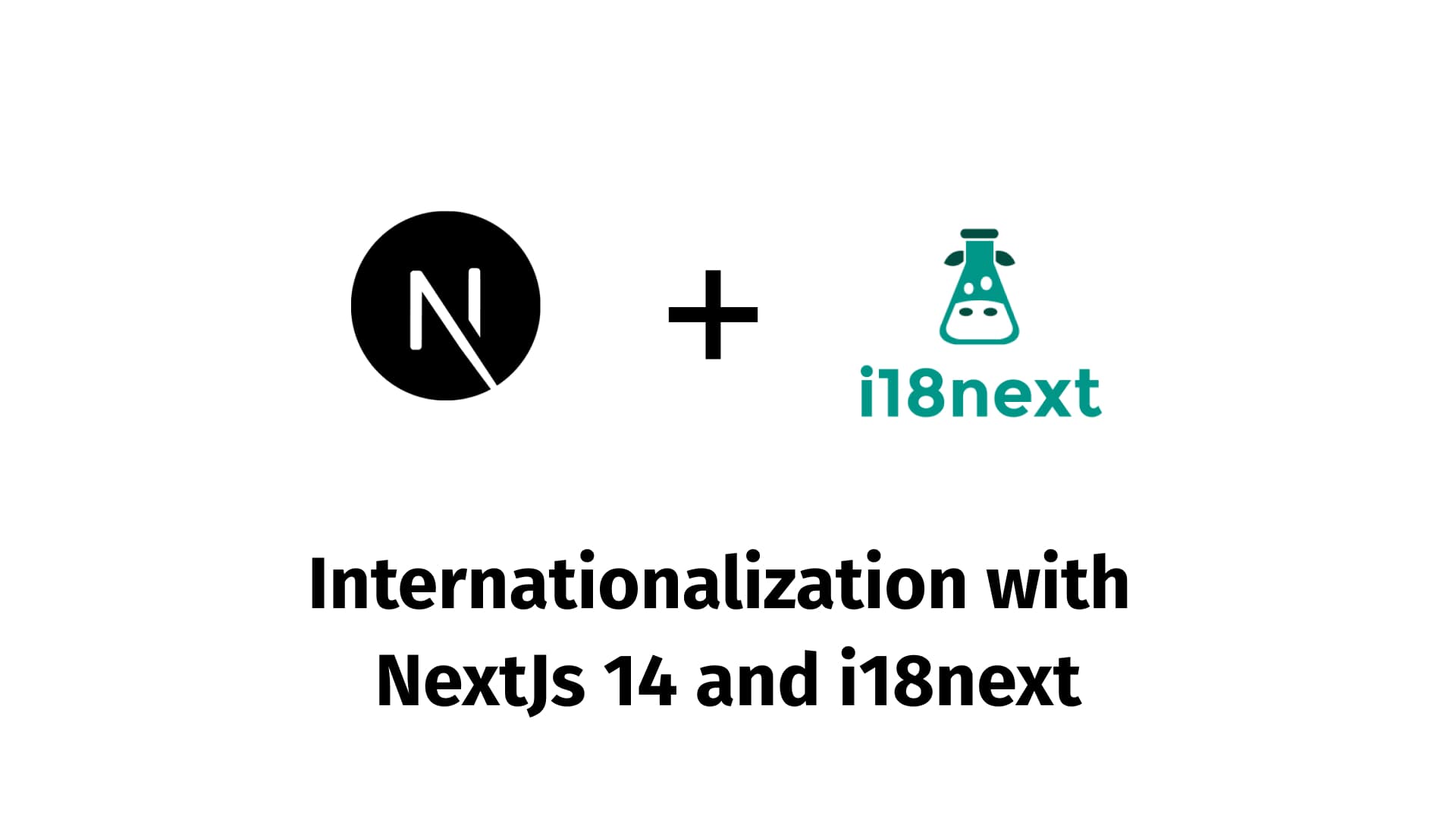
Introduction
Creating a multi-language app is essential for reaching a global audience and enhancing user experience. Next.js, a popular React framework, paired with i18next, a powerful internationalization library, makes building a multilingual application straightforward and efficient. In this guide, we'll walk through setting up a multi-language app using Next.js's App Router and i18next, covering everything from configuration to deployment.
Part 1: Setting Up Your Next.js Project
First, we need to create a new Next.js project. Open your terminal and run:
bun x create-next-app@latest my-multilingual-app
Navigate to your project directory:
cd my-multilingual-app
Part 2: Installing i18next and Required Packages
Install the necessary packages for internationalization:
bun add next-i18next i18next react-i18next
Part 3: Configuring i18next
To integrate i18next with Next.js, follow these steps:
-
Create Configuration Files
Create a new file named
next-i18next.config.js
in the root of your project:// next-i18next.config.js module.exports = { i18n: { defaultLocale: 'en', locales: ['en', 'fr', 'es'], // Add more locales as needed }, reloadOnPrerender: process.env.NODE_ENV === 'development', };
-
Update Next.js Configuration
Modify your
next.config.mjs
file to include i18next configuration:// next.config.mjs import { defineConfig } from 'next'; import NextI18Next from 'next-i18next'; import nextI18NextConfig from './next-i18next.config'; export default defineConfig({ i18n: nextI18NextConfig.i18n, });
Part 4: Adding Translation Files
Create a public/locales
directory and add translation files for each locale. For example, create en/translation.json
, fr/translation.json
, and es/translation.json
:
-
English Translation
// public/locales/en/translation.json { "welcome": "Welcome to our website!", "description": "This is a multi-language app built with Next.js and i18next." }
-
French Translation
// public/locales/fr/translation.json { "welcome": "Bienvenue sur notre site Web!", "description": "Ceci est une application multilingue construite avec Next.js et i18next." }
-
Spanish Translation
// public/locales/es/translation.json { "welcome": "¡Bienvenido a nuestro sitio web!", "description": "Esta es una aplicación multilingüe construida con Next.js y i18next." }
Part 5: Using Translations in Components
To use translations in your components, import useTranslation
from react-i18next
and use it to get translated strings:
-
Home Page Example
Create a
page.tsx
file in theapp
directory:// app/page.tsx import { useTranslation } from 'react-i18next'; export default function Home() { const { t } = useTranslation(); return ( <div> <h1>{t('welcome')}</h1> <p>{t('description')}</p> </div> ); }
Part 6: Adding Locale Switching
To allow users to switch between languages, you can add a language switcher component. Create a new component named LanguageSwitcher.tsx
:
// components/LanguageSwitcher.tsx
import { useRouter } from 'next/router';
import { useTranslation } from 'react-i18next';
const LanguageSwitcher = () => {
const { i18n } = useTranslation();
const router = useRouter();
const handleChange = (event: React.ChangeEvent<HTMLSelectElement>) => {
const locale = event.target.value;
router.push(router.asPath, router.asPath, { locale });
};
return (
<select value={i18n.language} onChange={handleChange}>
<option value="en">English</option>
<option value="fr">Français</option>
<option value="es">Español</option>
</select>
);
};
export default LanguageSwitcher;
Import and use the LanguageSwitcher
component in your layout or pages.
Part 7: Deploying Your Multi-language App
Deploying a Next.js app with internationalization is similar to deploying any other Next.js application. Ensure that your deployment platform supports server-side rendering and correctly handles locales. Platforms like Vercel and Netlify offer excellent support for Next.js apps.
Conclusion
Building a multi-language app with Next.js and i18next allows you to create a web application that caters to a global audience, enhancing user experience and accessibility. By following this guide, you’ve set up a robust internationalization framework, allowing users to easily switch languages and enjoy localized content.
Happy coding, and enjoy building multilingual applications with Next.js and i18next!