Building Real-time Applications with WebSockets and Next.js
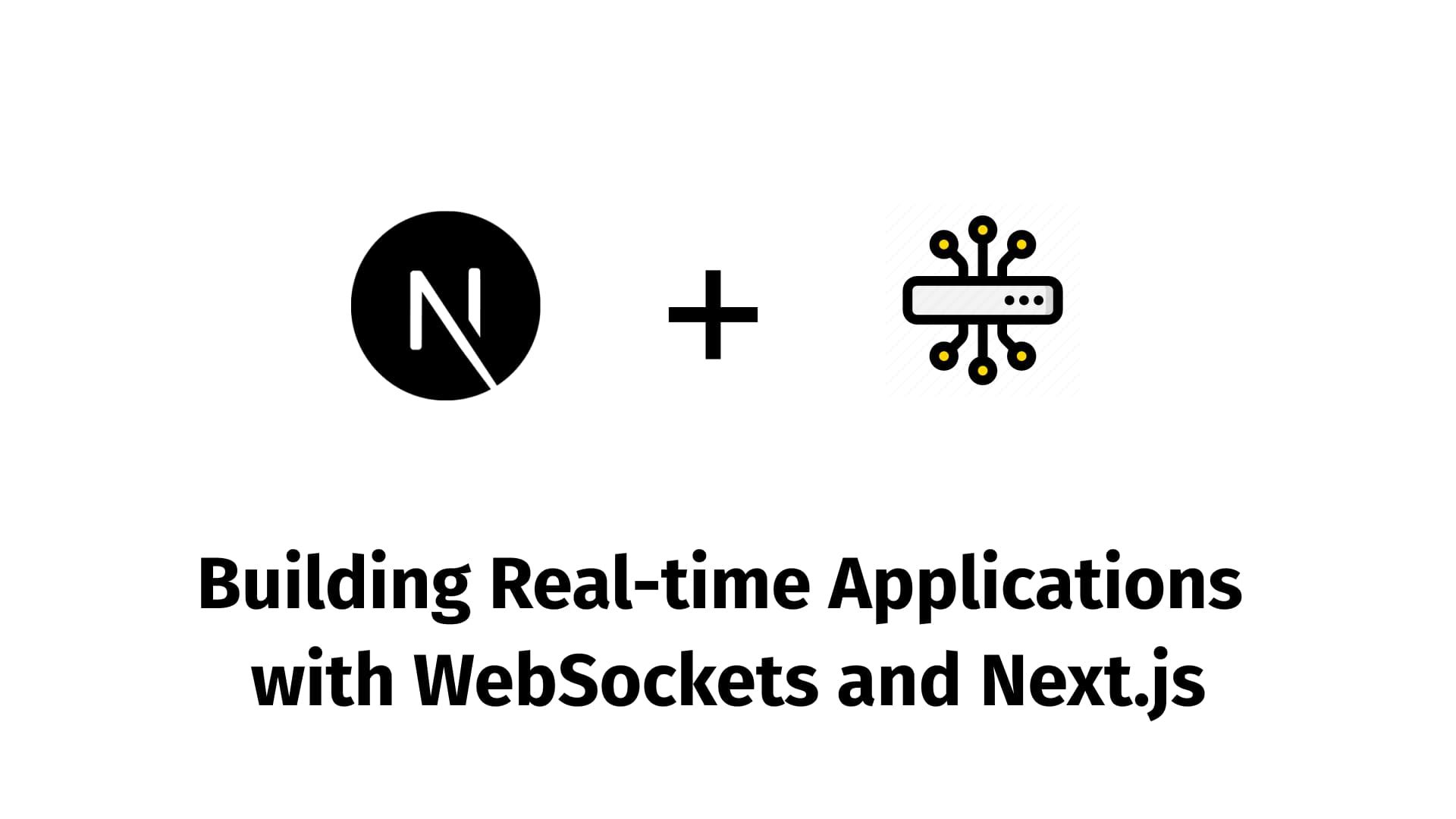
Introduction
Building real-time applications involves creating a seamless and interactive user experience with instant updates and data synchronization. WebSockets provide a robust solution for real-time communication between the client and server. When combined with Next.js and its App Router, you can efficiently handle real-time updates while leveraging Next.js’s powerful features for routing and data management. In this guide, we’ll walk through the process of building a real-time application using WebSockets and Next.js.
Part 1: Setting Up Your Next.js Project
First, ensure you have a Next.js project set up with the App Router. If you don’t already have one, create a new Next.js project using Bun:
bun x create-next-app@latest my-real-time-app
cd my-real-time-app
Part 2: Integrating WebSockets
To enable real-time communication, we’ll use the ws
library for WebSocket functionality. Start by installing the ws
package:
bun add ws
Create a WebSocket server to handle real-time connections:
-
Setting Up WebSocket Server
Create a file named
websocket-server.js
in your project root:// websocket-server.js const WebSocket = require('ws'); const server = new WebSocket.Server({ port: 8080 }); server.on('connection', (socket) => { console.log('New client connected'); socket.on('message', (message) => { console.log(`Received message => ${message}`); // Broadcast message to all clients server.clients.forEach((client) => { if (client.readyState === WebSocket.OPEN) { client.send(message); } }); }); socket.on('close', () => { console.log('Client disconnected'); }); }); console.log('WebSocket server is running on ws://localhost:8080');
Run the WebSocket server:
node websocket-server.js
-
Connecting WebSocket Client
In your Next.js application, connect to the WebSocket server from a React component. For demonstration, create a new component in
app/components/Chat.tsx
:// app/components/Chat.tsx 'use client'; import { useEffect, useState } from 'react'; export default function Chat() { const [socket, setSocket] = useState<WebSocket | null>(null); const [messages, setMessages] = useState<string[]>([]); const [input, setInput] = useState<string>(''); useEffect(() => { const ws = new WebSocket('ws://localhost:8080'); ws.onmessage = (event) => { setMessages((prev) => [...prev, event.data]); }; setSocket(ws); return () => ws.close(); }, []); const sendMessage = () => { if (socket) { socket.send(input); setInput(''); } }; return ( <div> <div> {messages.map((msg, index) => ( <div key={index}>{msg}</div> ))} </div> <input type="text" value={input} onChange={(e) => setInput(e.target.value)} /> <button onClick={sendMessage}>Send</button> </div> ); }
Part 3: Using the App Router for Real-Time Updates
Next.js's App Router allows you to manage and render routes efficiently. Here’s how to integrate the Chat
component into your application using the App Router:
-
Create a Route for Chat
Update
app/page.tsx
to include theChat
component:// app/page.tsx import Chat from '@/components/Chat'; export default function HomePage() { return ( <div> <h1>Real-Time Chat Application</h1> <Chat /> </div> ); }
-
Handle Dynamic Updates
The
Chat
component handles real-time updates by connecting to the WebSocket server and updating the UI accordingly. Ensure that your component efficiently manages state and updates to reflect real-time changes.
Part 4: Enhancing the Real-Time Experience
-
Error Handling
Add error handling to manage connection issues and provide feedback to users:
// app/components/Chat.tsx useEffect(() => { const ws = new WebSocket('ws://localhost:8080'); ws.onmessage = (event) => { setMessages((prev) => [...prev, event.data]); }; ws.onerror = (error) => { console.error('WebSocket Error:', error); }; setSocket(ws); return () => ws.close(); }, []);
-
Authentication and Security
For a production application, consider adding authentication to WebSocket connections and ensuring secure data transmission. Use
wss://
for secure WebSocket connections if deploying over HTTPS.
Conclusion
Building real-time applications with WebSockets and Next.js provides a dynamic and interactive user experience. By integrating WebSocket functionality with the App Router, you can manage real-time data efficiently and offer a seamless experience to your users. From setting up the WebSocket server to connecting the client and handling updates, this guide covers the essentials of creating real-time applications with Next.js. Happy coding!