Enhancing User Experience with Progressive Web Apps (PWAs) in Next.js
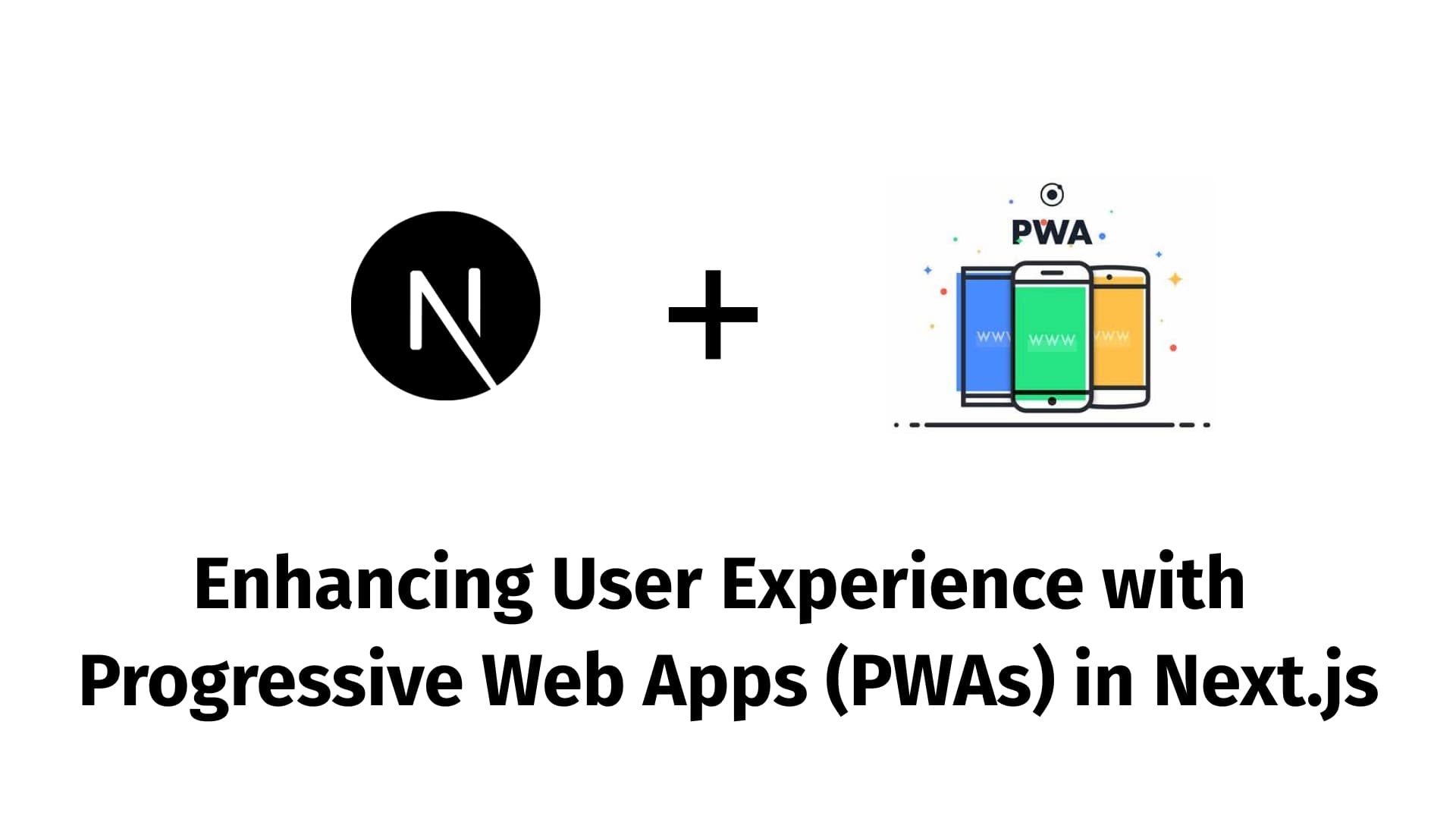
Introduction
Progressive Web Apps (PWAs) have revolutionized web development by combining the best features of web and mobile applications. They offer a native app-like experience with offline support, push notifications, and fast performance. Next.js, with its robust framework and modern features, is an excellent choice for building PWAs. In this guide, we’ll walk you through setting up a PWA in Next.js to enhance user experience and deliver a reliable, engaging web app.
Part 1: Setting Up Your Next.js Project
Start by creating a new Next.js project if you haven't already:
bun x create-next-app@latest my-pwa-app
Navigate to your project directory:
cd my-pwa-app
Part 2: Installing Required Packages
To turn your Next.js app into a Progressive Web App, you'll need a few additional packages:
bun add next-pwa
Part 3: Configuring next-pwa
The next-pwa
package makes it easy to add PWA features to your Next.js project. Configure it by creating or updating your next.config.mjs
file:
-
Configure
next.config.mjs
// next.config.mjs import NextPwa from 'next-pwa'; import { defineConfig } from 'next'; export default defineConfig({ pwa: { dest: 'public', register: true, skipWaiting: true, }, });
-
Create a Service Worker
The
next-pwa
package automatically generates a service worker, but you need to add a manifest file for PWA features.
Part 4: Creating a Web App Manifest
Create a manifest.json
file in the public
directory. This file contains metadata about your PWA:
// public/manifest.json
{
"name": "My PWA App",
"short_name": "PWA App",
"description": "A Progressive Web App built with Next.js",
"icons": [
{
"src": "/icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "/icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
],
"start_url": "/",
"display": "standalone",
"theme_color": "#000000",
"background_color": "#ffffff"
}
Make sure to add appropriate icons for your application in the public/icons
directory.
Part 5: Implementing Offline Support
The next-pwa
package handles most of the work for offline support by setting up a service worker. To further customize it, you can create a service-worker.js
file in the public
directory if you need special functionality.
Part 6: Adding PWA Features
-
Adding a Service Worker Script
If you want to customize your service worker, create a
service-worker.js
file in thepublic
directory:// public/service-worker.js self.addEventListener('install', (event) => { console.log('Service worker installing...'); // Cache assets or perform other install tasks }); self.addEventListener('fetch', (event) => { console.log('Fetching:', event.request.url); // Handle fetch events });
-
Testing Your PWA
You can test your PWA using Chrome DevTools. Go to the "Application" tab and check if your app is registered as a service worker and if the manifest is loaded.
Part 7: Enhancing User Experience
-
Push Notifications
Implement push notifications to keep users engaged even when they are not actively using your app. You can use the Web Push API or libraries like
web-push
to integrate notifications. -
Add a Home Screen Icon
PWAs allow users to add your app to their home screen, providing a native-like experience. Ensure your
manifest.json
includes theicons
field with appropriate sizes. -
Optimize Performance
Ensure that your app loads quickly and responds promptly to user interactions. Use techniques like lazy loading and code splitting to enhance performance.
Conclusion
By following this guide, you’ve transformed your Next.js application into a Progressive Web App, enhancing user experience with offline capabilities, push notifications, and a native-like interface. PWAs provide a seamless experience that can significantly improve user engagement and retention.
Deploy your PWA and enjoy the benefits of a fast, reliable, and engaging web application!