Mastering Static Site Generation with Next.js: Best Practices and Tips
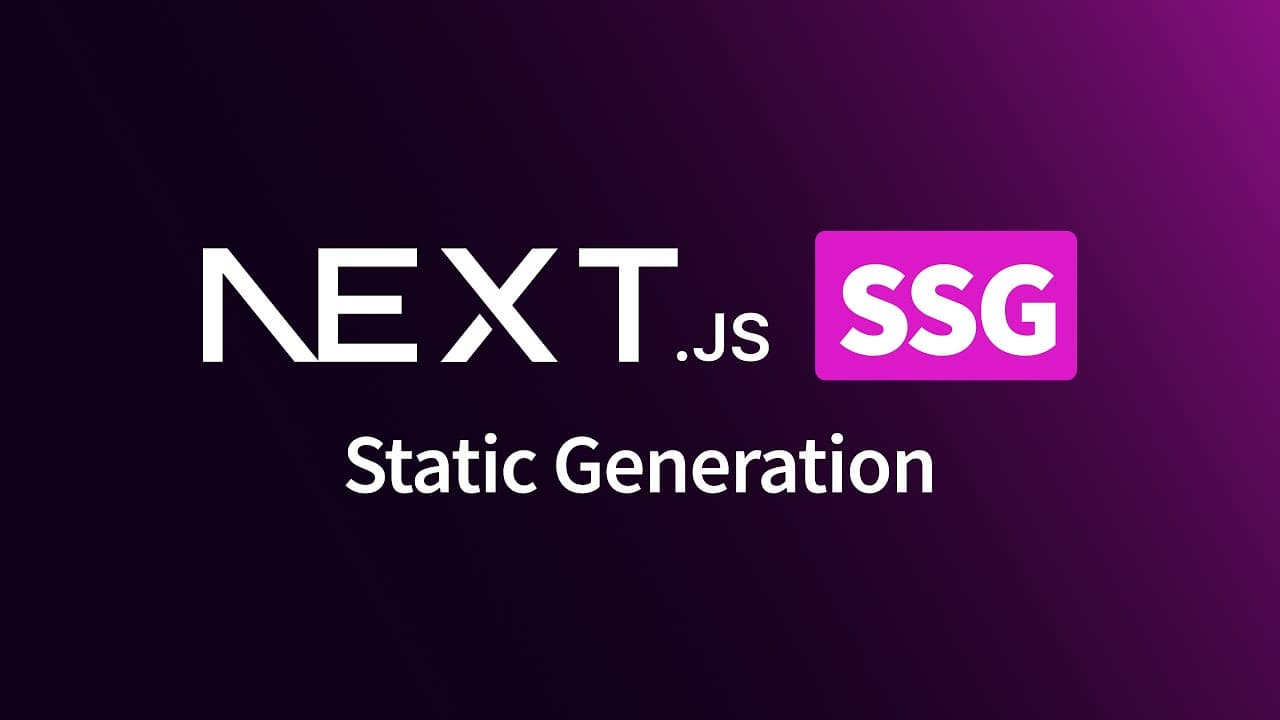
Next.js 14 offers a powerful Static Site Generation (SSG) feature that enables developers to generate static HTML at build time, delivering a fast, SEO-friendly experience for users. In this guide, we’ll cover some best practices and tips to fully utilize SSG in Next.js 14 and optimize your applications.
Step 1: Understanding Static Site Generation in Next.js 14
Static Site Generation allows you to generate HTML during build time for better performance and SEO. In Next.js 14, the primary method for creating static pages remains getStaticProps
, but it comes with performance improvements under the hood, offering faster build times and enhanced support for edge rendering.
Here’s how to use it:
export async function getStaticProps() {
const data = await fetchSomeData();
return {
props: {
data,
},
};
}
export default function Home({ data }) {
return (
<div>
<h1>Welcome to my website</h1>
<p>{data}</p>
</div>
);
}
By using getStaticProps
, you ensure that the data is fetched at build time, allowing your page to be served as static HTML for quicker loading times and improved SEO.
Step 2: Pre-rendering Pages with getStaticPaths
If you need to pre-render dynamic routes, getStaticPaths
works in conjunction with getStaticProps
. This is especially useful when generating pages for blogs, product listings, or other content-heavy websites.
Here’s an example:
export async function getStaticPaths() {
const posts = await fetchAllPosts();
const paths = posts.map((post) => ({
params: { id: post.id },
}));
return {
paths,
fallback: false,
};
}
export async function getStaticProps({ params }) {
const post = await fetchPostById(params.id);
return {
props: {
post,
},
};
}
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
}
In Next.js 14, getStaticPaths
remains essential for pre-rendering dynamic routes, ensuring that these pages are built at compile time for optimal performance.
Step 3: Leveraging ISR (Incremental Static Regeneration)
With Next.js 14, Incremental Static Regeneration (ISR) is further optimized for efficiency, allowing you to update static content without a full rebuild. You can specify revalidation intervals using the revalidate
key within getStaticProps
to control how often a page should be updated.
export async function getStaticProps() {
const data = await fetchSomeData();
return {
props: {
data,
},
revalidate: 60, // Revalidate every 60 seconds
};
}
In Next.js 14, ISR improvements provide even faster regeneration, helping your app stay fresh without compromising performance.
Step 4: Optimizing Your Site for SEO
With Static Site Generation in Next.js 14, SEO benefits are immense because search engines can crawl and index static HTML efficiently. To further optimize your site for SEO, follow these best practices:
- Use descriptive and meaningful
<meta>
tags for better search engine understanding. - Generate dynamic sitemaps for easier crawling by search bots.
- Use
next/head
to manage meta tags dynamically and enhance SEO on every page. - Improve load times by minimizing JavaScript and CSS bundles, utilizing Next.js 14’s built-in optimization features.
import Head from 'next/head';
export default function Home() {
return (
<div>
<Head>
<title>Welcome to My Next.js 14 Site</title>
<meta name="description" content="Learn more about Next.js 14 and SSG." />
</Head>
<h1>Welcome to my website</h1>
</div>
);
}
By adhering to these practices, you can ensure better visibility and ranking for your Next.js 14-powered website.
Conclusion
Static Site Generation in Next.js 14 offers a perfect balance of performance and scalability. When combined with best practices like optimizing for SEO and leveraging ISR, your projects will benefit from faster load times, enhanced user experiences, and better search engine rankings.
By fully embracing SSG in Next.js 14, you can build faster, more scalable websites and applications, ensuring your users receive the best possible experience.