Optimizing Next.js Performance: Techniques for Faster Load Times and Improved SEO
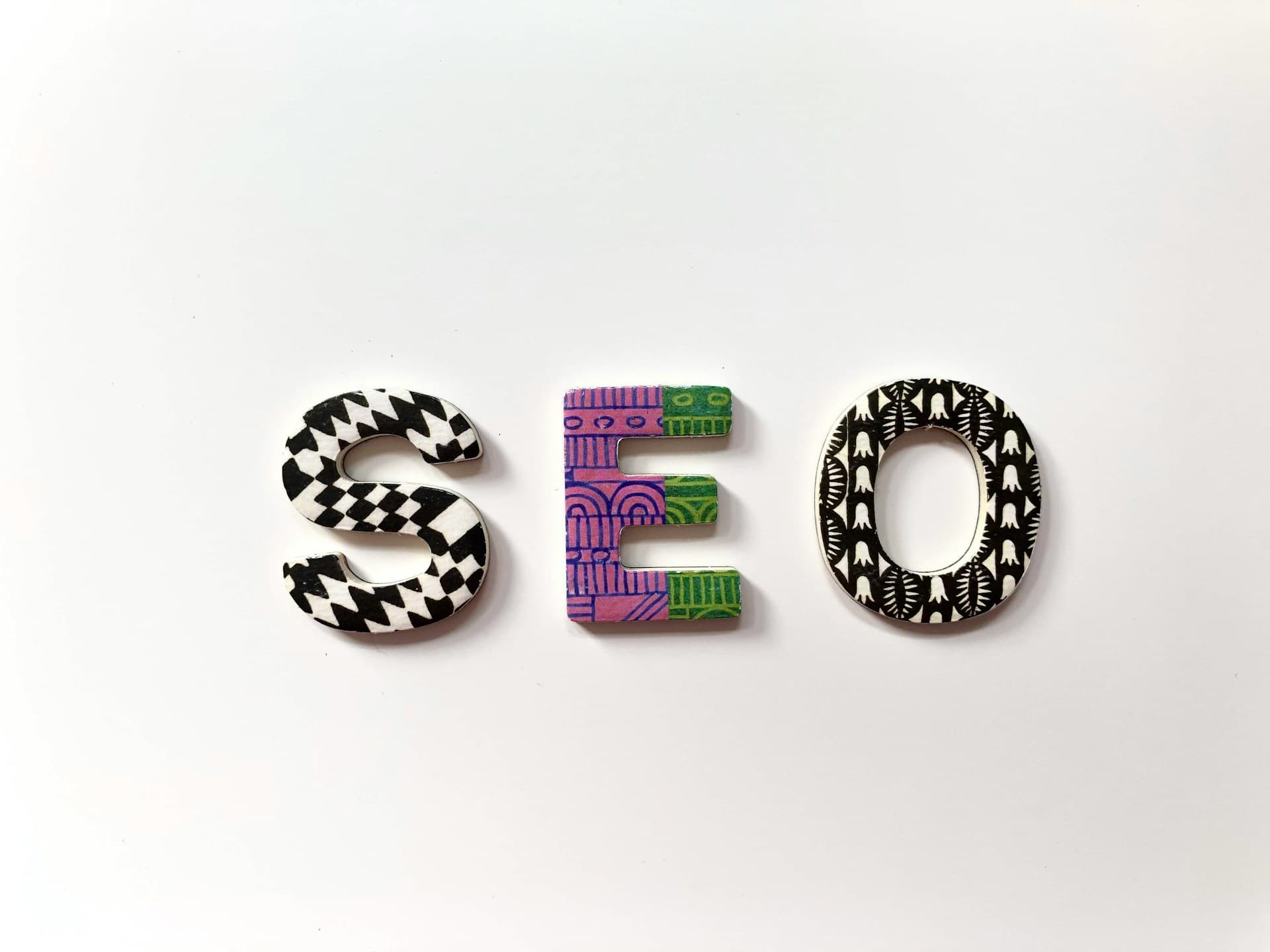
Introduction
Performance optimization is crucial for enhancing user experience and improving SEO in Next.js applications. With its built-in features and the App Router, Next.js offers various techniques to achieve faster load times and better search engine rankings. In this guide, we'll explore key strategies for optimizing your Next.js application, focusing on efficient rendering, code splitting, and SEO best practices.
Part 1: Efficient Rendering with Next.js App Router
The Next.js App Router is a powerful feature that helps manage routing and rendering efficiently. Here's how to leverage it for performance optimization:
-
Static Site Generation (SSG)
Use Static Site Generation to pre-render pages at build time. This technique ensures that users receive a fully-rendered HTML page quickly:
// app/page.tsx import { getStaticProps } from 'next'; export async function getStaticProps() { const data = await fetchData(); // Your data fetching logic return { props: { data } }; } export default function HomePage({ data }) { return ( <div> <h1>Home Page</h1> {/* Render your data */} </div> ); }
-
Incremental Static Regeneration (ISR)
ISR allows you to update static content after the site has been built. This is useful for pages with dynamic data:
// app/page.tsx export async function getStaticProps() { const data = await fetchData(); // Your data fetching logic return { props: { data }, revalidate: 10 }; // Revalidate every 10 seconds }
-
Server-Side Rendering (SSR)
For pages that need to be dynamically rendered on each request, use Server-Side Rendering:
// app/page.tsx import { GetServerSideProps } from 'next'; export const getServerSideProps: GetServerSideProps = async () => { const data = await fetchData(); // Your data fetching logic return { props: { data } }; }; export default function Page({ data }) { return ( <div> <h1>Dynamic Page</h1> {/* Render your data */} </div> ); }
Part 2: Code Splitting and Lazy Loading
Reducing the amount of JavaScript loaded initially can significantly speed up page load times. Implement code splitting and lazy loading to enhance performance:
-
Dynamic Imports
Use Next.js dynamic imports to load components only when they are needed:
// app/page.tsx import dynamic from 'next/dynamic'; const DynamicComponent = dynamic(() => import('@/components/DynamicComponent')); export default function Page() { return ( <div> <h1>Page with Dynamic Component</h1> <DynamicComponent /> </div> ); }
-
React.lazy and Suspense
For client-side rendering, use React's
lazy
andSuspense
to load components asynchronously:// app/page.tsx import React, { Suspense, lazy } from 'react'; const LazyComponent = lazy(() => import('@/components/LazyComponent')); export default function Page() { return ( <div> <h1>Page with Lazy Component</h1> <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> </div> ); }
Part 3: Optimizing Images and Static Assets
Efficient image and asset handling can greatly improve load times:
-
Next.js Image Component
Use the
next/image
component to automatically optimize images:// app/page.tsx import Image from 'next/image'; export default function Page() { return ( <div> <h1>Optimized Image</h1> <Image src="/path/to/image.jpg" alt="Description" width={500} height={300} /> </div> ); }
-
Static Asset Optimization
Store static assets like images and fonts in the
public
directory, and use caching strategies to serve them efficiently.
Part 4: Improving SEO with Next.js
Optimizing for search engines is essential for driving traffic to your application. Implement these SEO best practices:
-
Dynamic Metadata
Use Next.js's
Head
component to set dynamic metadata for each page:// app/page.tsx import Head from 'next/head'; export default function Page() { return ( <div> <Head> <title>Page Title</title> <meta name="description" content="Page description" /> <meta property="og:title" content="Page Title" /> <meta property="og:description" content="Page description" /> </Head> <h1>Page Content</h1> </div> ); }
-
Structured Data
Implement structured data using JSON-LD to help search engines understand the content of your pages:
// app/page.tsx import Head from 'next/head'; export default function Page() { return ( <div> <Head> <script type="application/ld+json" dangerouslySetInnerHTML={{ __html: JSON.stringify({ "@context": "https://schema.org", "@type": "WebPage", name: "Page Title", description: "Page description", }), }} /> </Head> <h1>Page Content</h1> </div> ); }
Conclusion
Optimizing performance and SEO in Next.js applications involves leveraging the App Router for efficient rendering, implementing code splitting and lazy loading, optimizing images and static assets, and following SEO best practices. By applying these techniques, you can ensure faster load times, a better user experience, and improved search engine rankings. With Next.js’s robust features and best practices, your application will be well-equipped to handle high traffic and provide a seamless experience to your users.