Server-Side Rendering with Next.js and Bun: The Ultimate Guide
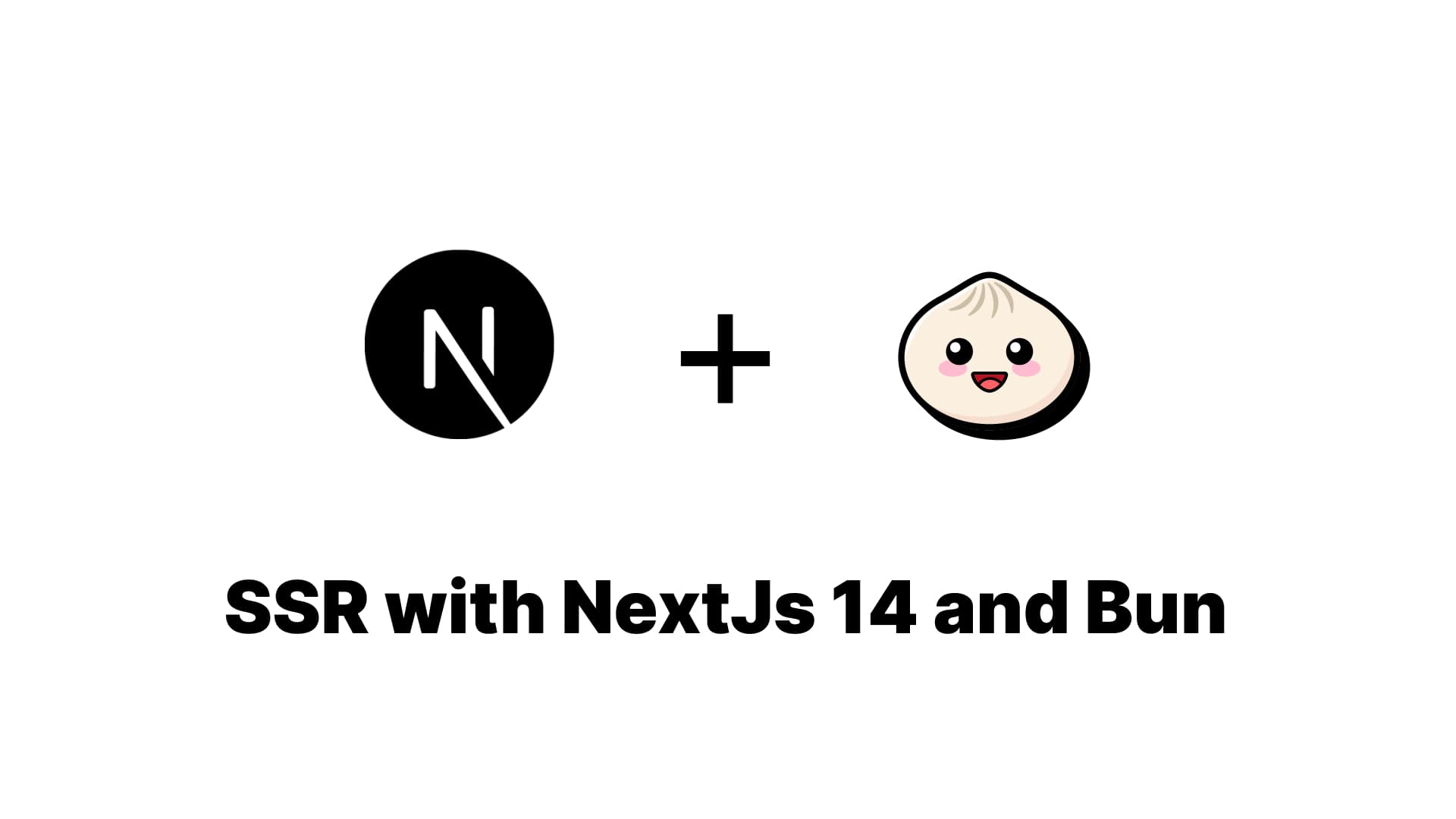
Introduction
Server-side rendering (SSR) has become a key feature for modern web applications, enhancing performance, SEO, and user experience. Next.js, a popular React framework, provides robust support for SSR out of the box. When combined with Bun, a modern JavaScript runtime, you can further optimize your SSR setup for speed and scalability. In this guide, we'll explore how to implement SSR in Next.js using Bun, offering practical tips and insights to get the most out of both technologies.
Part 1: Setting Up Your Next.js Project with Bun
Before diving into server-side rendering, let’s start by setting up a Next.js project with Bun. This process is straightforward and ensures that you have the latest tools at your disposal.
-
Create a Next.js Project
Start by creating a new Next.js project using Bun. Open your terminal and run:
bun x create-next-app@latest my-app
Navigate to your project directory:
cd my-app
-
Install Dependencies
Although Bun installs the necessary dependencies by default, ensure that you have the latest versions of required packages:
bun install
Part 2: Implementing Server-Side Rendering in Next.js
Next.js offers several ways to handle server-side rendering, including getServerSideProps
, getStaticProps
, and API routes. For this guide, we'll focus on getServerSideProps
, which is specifically used for SSR.
-
Creating a Page with SSR
To demonstrate SSR, let’s create a page that fetches data server-side and renders it. Create a new file in the
pages
directory calledssr-example.tsx
:// pages/ssr-example.tsx import { GetServerSideProps } from 'next'; interface Props { data: string; } const SSRExample: React.FC<Props> = ({ data }) => { return ( <div> <h1>Server-Side Rendered Page</h1> <p>Data fetched from the server: {data}</p> </div> ); }; export const getServerSideProps: GetServerSideProps<Props> = async () => { // Simulate fetching data from an API or database const data = await fetch('https://api.example.com/data') .then(res => res.json()) .then(json => json.message); return { props: { data } }; }; export default SSRExample;
This page will fetch data from an API on the server side and render it as HTML.
-
Testing the SSR Page
Start your Next.js development server:
bun dev
Open your browser and navigate to
http://localhost:3000/ssr-example
to see the server-side rendered content.
Part 3: Leveraging Bun for Enhanced Performance
Bun is designed to be a fast and modern JavaScript runtime that can improve various aspects of your development workflow, including SSR. Here’s how Bun enhances the SSR experience:
-
Fast Startup and Dependency Installation
Bun's fast startup time and efficient dependency management reduce the time it takes to start your server and install packages. This speed is beneficial during development and production builds.
-
Optimized Build Process
Bun offers an optimized build process that can result in faster server-side rendering times. Its approach to bundling and transpiling can reduce build times and enhance overall performance.
-
Debugging and Performance Insights
Use Bun's built-in tools and features to profile and debug your server-side rendering process. For example, you can leverage Bun’s efficient hot-reloading to see changes instantly without restarting your server.
Part 4: Debugging and Optimizing SSR Performance
Optimizing SSR performance involves several strategies to ensure that your application runs smoothly and efficiently.
-
Profiling Performance
Use performance profiling tools to identify bottlenecks in your SSR process. Analyze server response times and optimize your data fetching and rendering logic.
-
Caching Strategies
Implement caching strategies to reduce the load on your server. For example, you can use HTTP caching headers or a caching layer like Redis to store frequently accessed data.
-
Code Splitting and Optimization
Ensure that your application code is split and optimized to reduce the amount of JavaScript that needs to be processed during rendering. This approach helps in delivering faster initial page loads.
Conclusion
Server-side rendering with Next.js and Bun provides a powerful combination for building fast, scalable web applications. By leveraging the SSR capabilities of Next.js and the performance benefits of Bun, you can create applications that deliver excellent user experiences and perform optimally. Implementing the strategies discussed in this guide will help you harness the full potential of SSR and Bun.
Happy coding, and enjoy building with Next.js and Bun!