State Management in Next.js: A Deep Dive into Context API and Zustand
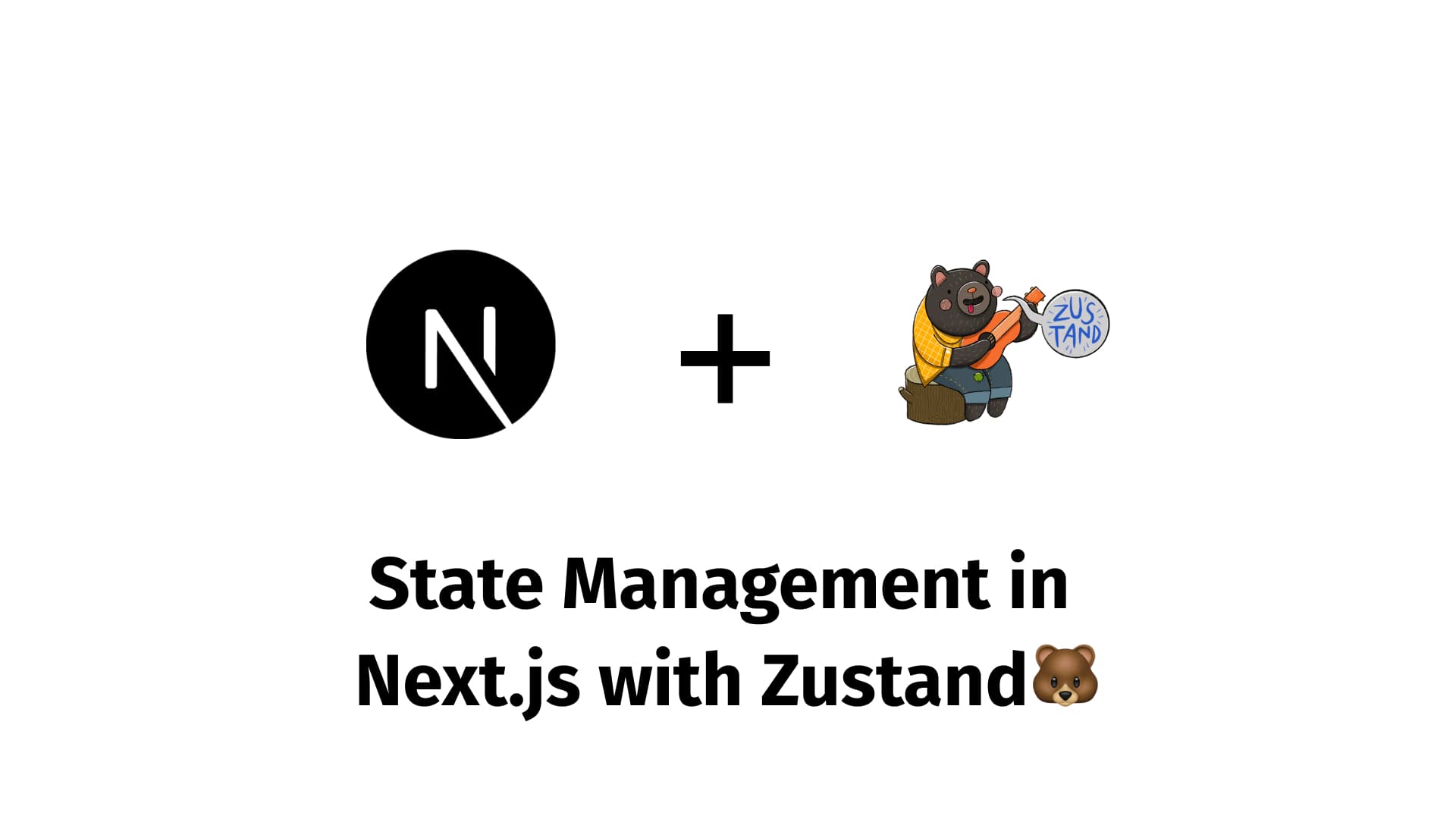
Introduction
Managing state effectively is crucial for building scalable and maintainable web applications. In Next.js, you have various options for state management, but two popular choices are the Context API and Zustand. Both have their own strengths and use cases, and in this blog, we'll dive deep into how to use these tools within a Next.js project.
Part 1: State Management with Context API
The Context API is a built-in feature of React that allows you to manage state across your component tree without passing props down manually. It’s especially useful for global state management, such as user authentication status or theme settings.
Setting Up Context API in Next.js
Let's set up a basic example where we manage user authentication status using the Context API.
-
Create a Context
Start by creating a context and a provider component. This provider will manage the authentication state.
// context/AuthContext.tsx import React, { createContext, useContext, useState, ReactNode } from 'react'; interface AuthContextType { isAuthenticated: boolean; login: () => void; logout: () => void; } const AuthContext = createContext<AuthContextType | undefined>(undefined); export const AuthProvider: React.FC<{ children: ReactNode }> = ({ children }) => { const [isAuthenticated, setIsAuthenticated] = useState<boolean>(false); const login = () => setIsAuthenticated(true); const logout = () => setIsAuthenticated(false); return ( <AuthContext.Provider value={{ isAuthenticated, login, logout }}> {children} </AuthContext.Provider> ); }; export const useAuth = () => { const context = useContext(AuthContext); if (context === undefined) { throw new Error('useAuth must be used within an AuthProvider'); } return context; };
-
Wrap Your Application with the Provider
Next, wrap your Next.js application with the
AuthProvider
so that the context is available throughout the app.// app/layout.tsx import { AuthProvider } from '@/context/AuthContext'; export default function RootLayout({ children }: { children: React.ReactNode }) { return ( <AuthProvider> {children} </AuthProvider> ); }
-
Consume the Context in Components
Finally, you can use the
useAuth
hook to access authentication state in your components.// app/page.tsx import { useAuth } from '@/context/AuthContext'; export default function Home() { const { isAuthenticated, login, logout } = useAuth(); return ( <div> <h1>{isAuthenticated ? 'Welcome Back!' : 'Please Log In'}</h1> {isAuthenticated ? ( <button onClick={logout}>Logout</button> ) : ( <button onClick={login}>Login</button> )} </div> ); }
Part 2: State Management with Zustand
Zustand is a small, fast, and scalable state management tool that can be used with React. It’s a good alternative to Context API for complex state management scenarios, providing a simple API and better performance.
Setting Up Zustand in Next.js
Let's create a state management store using Zustand to manage a counter in our application.
-
Install Zustand
First, install Zustand using Bun.
bun add zustand
-
Create a Store
Define a store to manage your state. Zustand stores are created using
create
function.// store/useCounterStore.ts import create from 'zustand'; interface CounterState { count: number; increment: () => void; decrement: () => void; } export const useCounterStore = create<CounterState>(set => ({ count: 0, increment: () => set(state => ({ count: state.count + 1 })), decrement: () => set(state => ({ count: state.count - 1 })), }));
-
Use the Store in Components
Now, use the Zustand store in your components to manage state.
// app/page.tsx import { useCounterStore } from '@/store/useCounterStore'; export default function Home() { const { count, increment, decrement } = useCounterStore(); return ( <div> <h1>Counter: {count}</h1> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); }
Part 3: Context API vs. Zustand
Both the Context API and Zustand have their use cases, and choosing between them depends on the complexity of your application:
-
Context API: Best suited for simple global state needs. It’s built into React and is ideal for scenarios where you need to pass down global data like authentication status or theme settings.
-
Zustand: Provides a more scalable solution for complex state management. It’s suitable for applications with intricate state logic, frequent state updates, or when you need a more performant alternative to Context API.
Conclusion
Understanding different state management solutions is key to building robust Next.js applications. Context API and Zustand offer different approaches to managing state, each with its own advantages. By leveraging these tools effectively, you can ensure that your Next.js projects are both scalable and maintainable.
Happy coding, and choose the right tool for your state management needs!